Data Fetching and Caching
Examples
This guide will walk you through the basics of data fetching and caching in Next.js, providing practical examples and best practices.
Here's a minimal example of data fetching in Next.js:
export default async function Page() {
let data = await fetch('https://api.vercel.app/blog')
let posts = await data.json()
return (
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)
}
This example demonstrates a basic server-side data fetch using the fetch
API in an asynchronous React Server Component.
Reference
fetch
- React
cache
- Next.js
unstable_cache
Examples
Fetching data on the server with the fetch
API
This component will fetch and display a list of blog posts. The response from fetch
will be automatically cached.
export default async function Page() {
let data = await fetch('https://api.vercel.app/blog')
let posts = await data.json()
return (
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)
}
If you are not using any dynamic functions anywhere else in this route, it will be prerendered during next build
to a static page. The data can then be updated using Incremental Static Regeneration.
If you do not want to cache the response from fetch
, you can do the following:
let data = await fetch('https://api.vercel.app/blog', { cache: 'no-store' })
Fetching data on the server with an ORM or database
This component will fetch and display a list of blog posts. The response from the database is not cached by default but could be with additional configuration.
import { db, posts } from '@/lib/db'
export default async function Page() {
let allPosts = await db.select().from(posts)
return (
<ul>
{allPosts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)
}
If you are not using any dynamic functions anywhere else in this route, it will be prerendered during next build
to a static page. The data can then be updated using Incremental Static Regeneration.
To prevent the page from prerendering, you can add the following to your file:
export const dynamic = 'force-dynamic'
However, you will commonly use functions like cookies()
, headers()
, or reading the incoming searchParams
from the page props, which will automatically make the page render dynamically. In this case, you do not need to explicitly use force-dynamic
.
Fetching data on the client
We recommend first attempting to fetch data on the server-side.
However, there are still cases where client-side data fetching makes sense. In these scenarios, you can manually call fetch
in a useEffect
(not recommended), or lean on popular React libraries in the community (such as SWR or React Query) for client fetching.
'use client'
import { useState, useEffect } from 'react'
export function Posts() {
const [posts, setPosts] = useState(null)
useEffect(() => {
async function fetchPosts() {
let res = await fetch('https://api.vercel.app/blog')
let data = await res.json()
setPosts(data)
}
fetchPosts()
}, [])
if (!posts) return <div>Loading...</div>
return (
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)
}
Caching data with an ORM or Database
You can use the unstable_cache
API to cache the response to allow pages to be prerendered when running next build
.
import { unstable_cache } from 'next/cache'
import { db, posts } from '@/lib/db'
const getPosts = unstable_cache(
async () => {
return await db.select().from(posts)
},
['posts'],
{ revalidate: 3600, tags: ['posts'] }
)
export default async function Page() {
const allPosts = await getPosts()
return (
<ul>
{allPosts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)
}
This example caches the result of the database query for 1 hour (3600 seconds). It also adds the cache tag posts
which can then be invalidated with Incremental Static Regeneration.
Reusing data across multiple functions
Next.js uses APIs like generateMetadata
and generateStaticParams
where you will need to use the same data fetched in the page
.
If you are using fetch
, requests are automatically memoized. This means you can safely call the same URL with the same options, and only one request will be made.
import { notFound } from 'next/navigation'
interface Post {
id: string
title: string
content: string
}
async function getPost(id: string) {
let res = await fetch(`https://api.vercel.app/blog/${id}`)
let post: Post = await res.json()
if (!post) notFound()
return post
}
export async function generateStaticParams() {
let posts = await fetch('https://api.vercel.app/blog').then((res) =>
res.json()
)
return posts.map((post: Post) => ({
id: post.id,
}))
}
export async function generateMetadata({ params }: { params: { id: string } }) {
let post = await getPost(params.id)
return {
title: post.title,
}
}
export default async function Page({ params }: { params: { id: string } }) {
let post = await getPost(params.id)
return (
<article>
<h1>{post.title}</h1>
<p>{post.content}</p>
</article>
)
}
If you are not using fetch
, and instead using an ORM or database directly, you can wrap your data fetch with the React cache
function. This will de-duplicate and only make one query.
import { cache } from 'react'
import { db, posts, eq } from '@/lib/db' // Example with Drizzle ORM
import { notFound } from 'next/navigation'
export const getPost = cache(async (id) => {
const post = await db.query.posts.findFirst({
where: eq(posts.id, parseInt(id)),
})
if (!post) notFound()
return post
})
Revalidating cached data
Learn more about revalidating cached data with Incremental Static Regeneration.
Patterns
Parallel and sequential data fetching
When fetching data inside components, you need to be aware of two data fetching patterns: Parallel and Sequential.

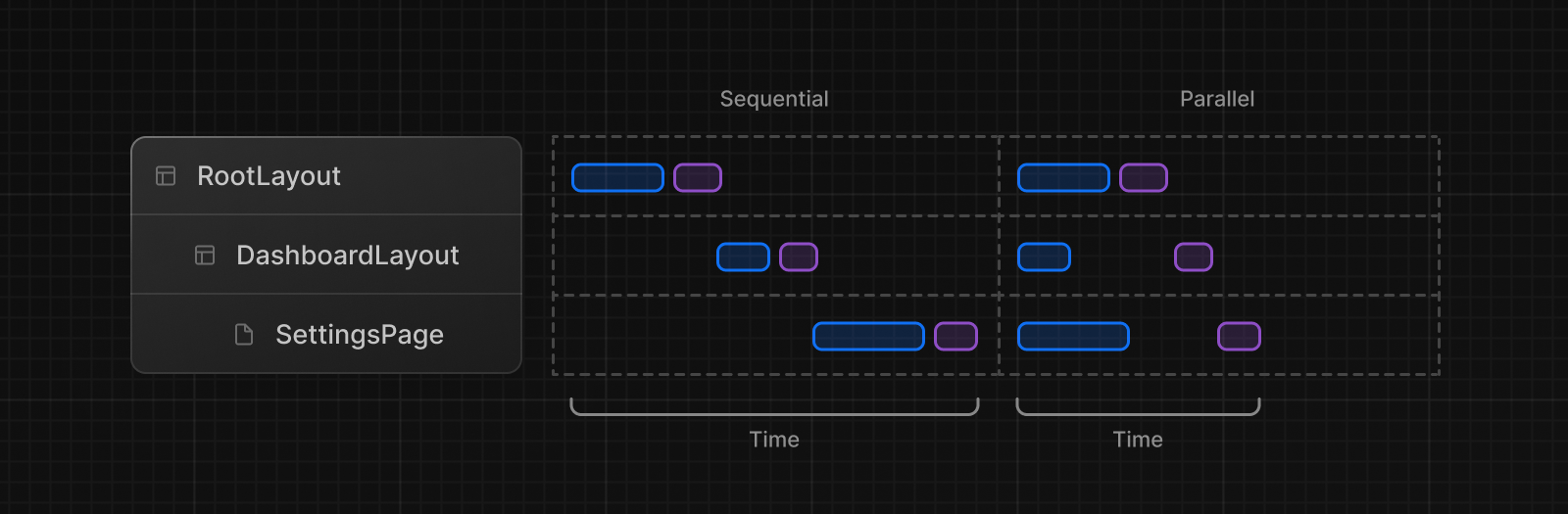
- Sequential: requests in a component tree are dependent on each other. This can lead to longer loading times.
- Parallel: requests in a route are eagerly initiated and will load data at the same time. This reduces the total time it takes to load data.
Sequential data fetching
If you have nested components, and each component fetches its own data, then data fetching will happen sequentially if those data requests are not memoized.
There may be cases where you want this pattern because one fetch depends on the result of the other. For example, the Playlists
component will only start fetching data once the Artist
component has finished fetching data because Playlists
depends on the artistID
prop:
export default async function Page({
params: { username },
}: {
params: { username: string }
}) {
// Get artist information
const artist = await getArtist(username)
return (
<>
<h1>{artist.name}</h1>
{/* Show fallback UI while the Playlists component is loading */}
<Suspense fallback={<div>Loading...</div>}>
{/* Pass the artist ID to the Playlists component */}
<Playlists artistID={artist.id} />
</Suspense>
</>
)
}
async function Playlists({ artistID }: { artistID: string }) {
// Use the artist ID to fetch playlists
const playlists = await getArtistPlaylists(artistID)
return (
<ul>
{playlists.map((playlist) => (
<li key={playlist.id}>{playlist.name}</li>
))}
</ul>
)
}
You can use loading.js
(for route segments) or React <Suspense>
(for nested components) to show an instant loading state while React streams in the result.
This will prevent the whole route from being blocked by data requests, and the user will be able to interact with the parts of the page that are ready.
Parallel Data Fetching
By default, layout and page segments are rendered in parallel. This means requests will be initiated in parallel.
However, due to the nature of async
/await
, an awaited request inside the same segment or component will block any requests below it.
To fetch data in parallel, you can eagerly initiate requests by defining them outside the components that use the data. This saves time by initiating both requests in parallel, however, the user won't see the rendered result until both promises are resolved.
In the example below, the getArtist
and getAlbums
functions are defined outside the Page
component and initiated inside the component using Promise.all
:
import Albums from './albums'
async function getArtist(username: string) {
const res = await fetch(`https://api.example.com/artist/${username}`)
return res.json()
}
async function getAlbums(username: string) {
const res = await fetch(`https://api.example.com/artist/${username}/albums`)
return res.json()
}
export default async function Page({
params: { username },
}: {
params: { username: string }
}) {
const artistData = getArtist(username)
const albumsData = getAlbums(username)
// Initiate both requests in parallel
const [artist, albums] = await Promise.all([artistData, albumsData])
return (
<>
<h1>{artist.name}</h1>
<Albums list={albums} />
</>
)
}
In addition, you can add a Suspense Boundary to break up the rendering work and show part of the result as soon as possible.
Preloading Data
Another way to prevent waterfalls is to use the preload pattern by creating an utility function that you eagerly call above blocking requests. For example, checkIsAvailable()
blocks <Item/>
from rendering, so you can call preload()
before it to eagerly initiate <Item/>
data dependencies. By the time <Item/>
is rendered, its data has already been fetched.
Note that preload
function doesn't block checkIsAvailable()
from running.
import { getItem } from '@/utils/get-item'
export const preload = (id: string) => {
// void evaluates the given expression and returns undefined
// https://developer.mozilla.org/docs/Web/JavaScript/Reference/Operators/void
void getItem(id)
}
export default async function Item({ id }: { id: string }) {
const result = await getItem(id)
// ...
}
import Item, { preload, checkIsAvailable } from '@/components/Item'
export default async function Page({
params: { id },
}: {
params: { id: string }
}) {
// starting loading item data
preload(id)
// perform another asynchronous task
const isAvailable = await checkIsAvailable()
return isAvailable ? <Item id={id} /> : null
}
Good to know: The "preload" function can also have any name as it's a pattern, not an API.
Using React cache
and server-only
with the Preload Pattern
You can combine the cache
function, the preload
pattern, and the server-only
package to create a data fetching utility that can be used throughout your app.
import { cache } from 'react'
import 'server-only'
export const preload = (id: string) => {
void getItem(id)
}
export const getItem = cache(async (id: string) => {
// ...
})
With this approach, you can eagerly fetch data, cache responses, and guarantee that this data fetching only happens on the server.
The utils/get-item
exports can be used by Layouts, Pages, or other components to give them control over when an item's data is fetched.
Good to know:
- We recommend using the
server-only
package to make sure server data fetching functions are never used on the client.
Preventing sensitive data from being exposed to the client
We recommend using React's taint APIs, taintObjectReference
and taintUniqueValue
, to prevent whole object instances or sensitive values from being passed to the client.
To enable tainting in your application, set the Next.js Config experimental.taint
option to true
:
module.exports = {
experimental: {
taint: true,
},
}
Then pass the object or value you want to taint to the experimental_taintObjectReference
or experimental_taintUniqueValue
functions:
import { queryDataFromDB } from './api'
import {
experimental_taintObjectReference,
experimental_taintUniqueValue,
} from 'react'
export async function getUserData() {
const data = await queryDataFromDB()
experimental_taintObjectReference(
'Do not pass the whole user object to the client',
data
)
experimental_taintUniqueValue(
"Do not pass the user's address to the client",
data,
data.address
)
return data
}
import { getUserData } from './data'
export async function Page() {
const userData = getUserData()
return (
<ClientComponent
user={userData} // this will cause an error because of taintObjectReference
address={userData.address} // this will cause an error because of taintUniqueValue
/>
)
}
Was this helpful?